Fetch isotopic images¶
[ ]:
%matplotlib inline
from matplotlib import pyplot as plt
import pandas as pd
import numpy as np
from metaspace import SMInstance
import getpass
sm = SMInstance()
sm
This notebook illustrates how to pull ion images for annotations from METASPACE. It will obtain and display all the isotope images for a set of annotations.
Enter your API Key (only required for private datasets)¶
To access private datasets on METASPACE, generate an API key from your account page and enter it when prompted below. This step can be skipped for accessing public datasets.
[ ]:
# This will prompt you to enter your API key if needed and it will save it to a config file.
# Note that API keys should be kept secret like passwords.
sm.save_login()
Analysis¶
Whilst the API does support access by dataset name, it’s most reliable to query using the dataset id. A quick hack to grab this through the web app is to filter the dataset then check the url:
The id for the selected dataset(s) can be copied from here e.g.
http.://metaspace2020.eu/#/annotations?ds=2016-09-22_11h16m17s&sort=-msm
[2]:
ds = sm.dataset(id="2016-09-22_11h16m17s")
results = ds.results()
annotations = results[results.fdr <= 0.1][['msm']]
annotations.head()
[2]:
msm | ||
---|---|---|
formula | adduct | |
C40H80NO8P | +K | 0.991456 |
C43H76NO7P | +Na | 0.987877 |
C42H84NO8P | +K | 0.987600 |
C37H71O8P | +K | 0.987128 |
C44H86NO8P | +K | 0.976368 |
Download and plot all isotopic images for the first 5 annotations¶
[3]:
limit = 5 # number of annotations to get
plt.figure(figsize=(20, 16))
for ii in range(limit):
row = results.iloc[ii]
(sf, adduct) = row.name
images = ds.isotope_images(sf, adduct)
for j, im in enumerate(images):
plt.subplot(limit, 4, ii * 4 + j + 1)
plt.title("[{}{}]+ m/z {}".format(sf, adduct, images.peak(index=j)))
plt.imshow(images[j], cmap='viridis')
plt.show()
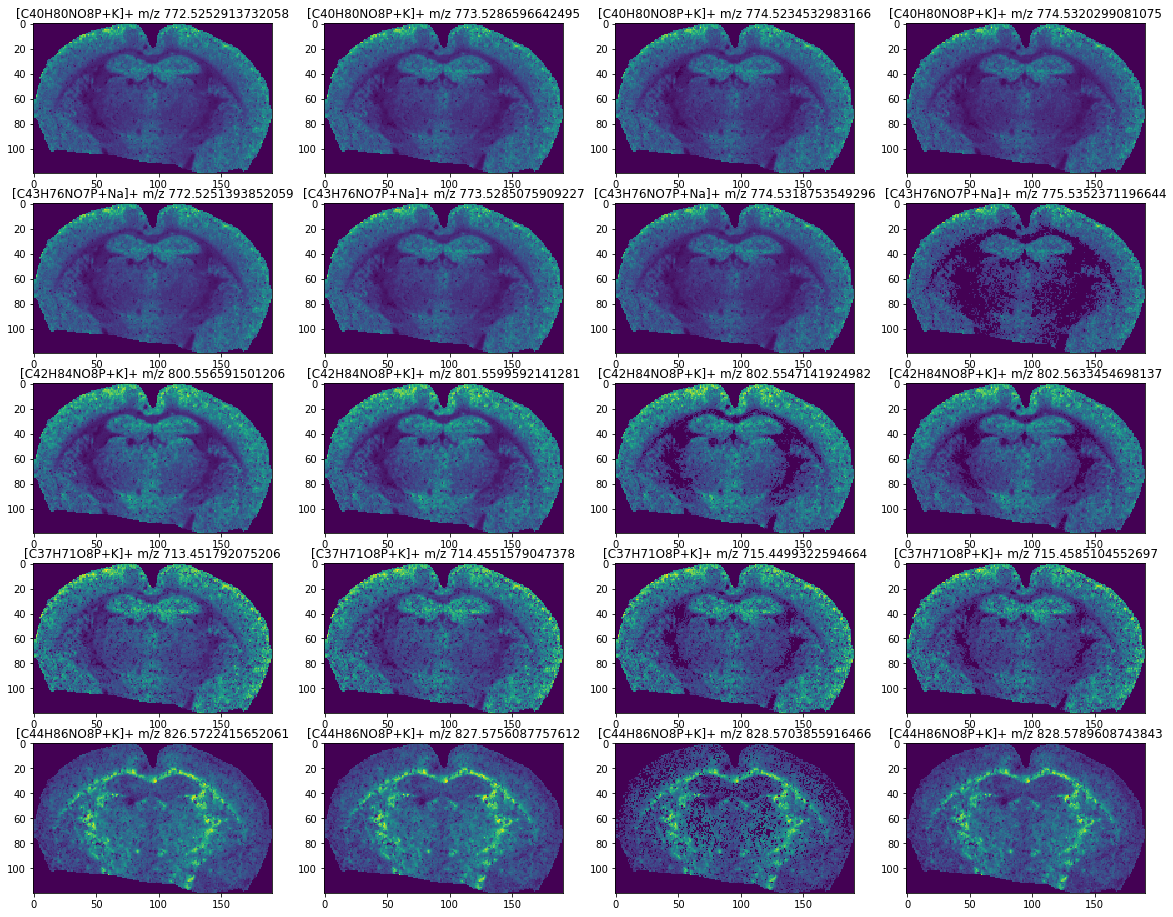
Bulk-download one image per annotation and calculate the pearson correlation between images¶
[4]:
isotope_image_sets = ds.all_annotation_images(fdr=0.10, only_first_isotope=True, scale_intensity=False)
# Convert images into the format NumPy expects: a 2D array where each row
# is one annotation's pixels / each column is one pixel across all annotations
images = np.array([imgs[0].flatten() for imgs in isotope_image_sets])
labels = [(imgs.formula, imgs.adduct) for imgs in isotope_image_sets]
corr = np.corrcoef(images)
corr_df = pd.DataFrame(corr, index=labels, columns=labels)
corr_df
[4]:
(C40H80NO8P, +K) | (C43H76NO7P, +Na) | (C42H84NO8P, +K) | (C37H71O8P, +K) | (C44H86NO8P, +K) | (C41H83N2O6P, +K) | (C45H80NO7P, +Na) | (C40H80NO8P, +Na) | (C42H82NO8P, +K) | (C40H80NO8P, +H) | ... | (C20H38O2, +H) | (C43H84NO8P, +H) | (C42H78NO8P, +H) | (C44H82NO8P, +H) | (C43H87N2O6P, +K) | (C42H83O10P, +Na) | (C8H20NO6P, +K) | (C48H84NO8P, +K) | (C16H16O11S, +K) | (C45H78NO8P, +K) | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
(C40H80NO8P, +K) | 1.000000 | 1.000000 | 0.952897 | 0.967237 | 0.245976 | 0.833225 | 0.952896 | 0.982682 | 0.848139 | 0.921046 | ... | -0.007426 | 0.851782 | 0.982674 | 0.933620 | 0.382377 | 0.952055 | 0.152810 | 0.490398 | 0.289137 | 0.636388 |
(C43H76NO7P, +Na) | 1.000000 | 1.000000 | 0.952897 | 0.967237 | 0.245976 | 0.833225 | 0.952896 | 0.982682 | 0.848139 | 0.921046 | ... | -0.007426 | 0.851782 | 0.982674 | 0.933620 | 0.382377 | 0.952055 | 0.152810 | 0.490398 | 0.289137 | 0.636388 |
(C42H84NO8P, +K) | 0.952897 | 0.952897 | 1.000000 | 0.928603 | 0.265837 | 0.769453 | 1.000000 | 0.958757 | 0.843311 | 0.878954 | ... | -0.004923 | 0.818077 | 0.958749 | 0.976383 | 0.477978 | 0.998177 | 0.155859 | 0.561101 | 0.287036 | 0.665877 |
(C37H71O8P, +K) | 0.967237 | 0.967237 | 0.928603 | 1.000000 | 0.311083 | 0.826645 | 0.928601 | 0.959579 | 0.874272 | 0.867523 | ... | -0.006511 | 0.879146 | 0.959580 | 0.900842 | 0.381378 | 0.929519 | 0.212115 | 0.543898 | 0.413908 | 0.725554 |
(C44H86NO8P, +K) | 0.245976 | 0.245976 | 0.265837 | 0.311083 | 1.000000 | 0.315141 | 0.265828 | 0.282098 | 0.663409 | 0.230023 | ... | 0.008136 | 0.491154 | 0.282133 | 0.213727 | 0.259783 | 0.265818 | 0.740538 | 0.636748 | 0.721979 | 0.514660 |
(C41H83N2O6P, +K) | 0.833225 | 0.833225 | 0.769453 | 0.826645 | 0.315141 | 1.000000 | 0.769453 | 0.841931 | 0.799991 | 0.750975 | ... | -0.004867 | 0.801050 | 0.841924 | 0.760261 | 0.308824 | 0.766656 | 0.340360 | 0.448071 | 0.326168 | 0.593814 |
(C45H80NO7P, +Na) | 0.952896 | 0.952896 | 1.000000 | 0.928601 | 0.265828 | 0.769453 | 1.000000 | 0.958756 | 0.843308 | 0.878951 | ... | -0.004923 | 0.818067 | 0.958748 | 0.976380 | 0.477895 | 0.998177 | 0.155857 | 0.561080 | 0.287023 | 0.665875 |
(C40H80NO8P, +Na) | 0.982682 | 0.982682 | 0.958757 | 0.959579 | 0.282098 | 0.841931 | 0.958756 | 1.000000 | 0.856468 | 0.915175 | ... | -0.007160 | 0.859451 | 0.999993 | 0.955873 | 0.427682 | 0.957449 | 0.168905 | 0.517190 | 0.305991 | 0.645559 |
(C42H82NO8P, +K) | 0.848139 | 0.848139 | 0.843311 | 0.874272 | 0.663409 | 0.799991 | 0.843308 | 0.856468 | 1.000000 | 0.752610 | ... | 0.000489 | 0.889056 | 0.856479 | 0.780084 | 0.430843 | 0.843408 | 0.501314 | 0.777492 | 0.593308 | 0.833599 |
(C40H80NO8P, +H) | 0.921046 | 0.921046 | 0.878954 | 0.867523 | 0.230023 | 0.750975 | 0.878951 | 0.915175 | 0.752610 | 1.000000 | ... | -0.000408 | 0.863759 | 0.915163 | 0.896570 | 0.369670 | 0.876900 | 0.058189 | 0.408694 | 0.193200 | 0.515406 |
(C36H75N, +H) | -0.009600 | -0.009600 | -0.006478 | -0.007373 | 0.011932 | -0.007958 | -0.006478 | -0.008536 | 0.001118 | -0.008468 | ... | 0.932312 | -0.004992 | -0.008534 | -0.008279 | -0.000601 | -0.007299 | 0.009374 | 0.008436 | 0.011921 | -0.006137 |
(C45H91N2O6P, +Na) | -0.052784 | -0.052784 | -0.066423 | -0.045096 | 0.051254 | -0.037897 | -0.066523 | -0.039045 | -0.035051 | -0.026981 | ... | -0.001376 | -0.001272 | -0.039039 | -0.048234 | 0.679391 | -0.067202 | 0.015372 | 0.046015 | 0.081648 | -0.064650 |
(C44H86NO8P, +H) | 0.245644 | 0.245644 | 0.244563 | 0.266167 | 0.853654 | 0.283919 | 0.244544 | 0.275143 | 0.547783 | 0.368546 | ... | 0.009352 | 0.519933 | 0.275162 | 0.244955 | 0.238411 | 0.243413 | 0.522728 | 0.414717 | 0.519774 | 0.297139 |
(C41H77O8P, +K) | 0.210762 | 0.210762 | 0.221701 | 0.299568 | 0.978221 | 0.284668 | 0.221688 | 0.247133 | 0.620025 | 0.197050 | ... | 0.008431 | 0.479948 | 0.247174 | 0.177315 | 0.229913 | 0.222255 | 0.737555 | 0.590756 | 0.764812 | 0.493705 |
(C44H86NO8P, +Na) | 0.374057 | 0.374057 | 0.394609 | 0.436303 | 0.955099 | 0.466513 | 0.394577 | 0.427371 | 0.741378 | 0.382486 | ... | 0.007935 | 0.629556 | 0.427405 | 0.365155 | 0.402833 | 0.394298 | 0.679352 | 0.667214 | 0.688418 | 0.572253 |
(C35H66O4, +H) | 0.913117 | 0.913117 | 0.883293 | 0.973004 | 0.303228 | 0.791940 | 0.883290 | 0.920476 | 0.830891 | 0.847750 | ... | -0.005603 | 0.897092 | 0.920478 | 0.874707 | 0.376022 | 0.885332 | 0.172918 | 0.526275 | 0.401639 | 0.727048 |
(C45H78NO7P, +Na) | 0.848139 | 0.848139 | 0.843311 | 0.874272 | 0.663409 | 0.799991 | 0.843308 | 0.856468 | 1.000000 | 0.752610 | ... | 0.000489 | 0.889056 | 0.856479 | 0.780084 | 0.430843 | 0.843408 | 0.501314 | 0.777492 | 0.593308 | 0.833599 |
(C42H84NO8P, +H) | 0.863766 | 0.863766 | 0.887750 | 0.801698 | 0.240099 | 0.672882 | 0.887751 | 0.876120 | 0.716219 | 0.955765 | ... | 0.002122 | 0.820121 | 0.876103 | 0.914706 | 0.430544 | 0.885567 | 0.054280 | 0.438330 | 0.166587 | 0.489774 |
(C45H91N2O6P, +H) | -0.046544 | -0.046544 | -0.057529 | -0.039530 | 0.046183 | -0.033571 | -0.057667 | -0.036520 | -0.029975 | -0.017688 | ... | -0.001129 | 0.006288 | -0.036515 | -0.039828 | 0.641025 | -0.058414 | 0.006987 | 0.045510 | 0.078993 | -0.055700 |
(C42H84NO8P, +Na) | 0.933645 | 0.933645 | 0.976458 | 0.900813 | 0.213180 | 0.760246 | 0.976458 | 0.955848 | 0.779990 | 0.896295 | ... | -0.006614 | 0.808984 | 0.955838 | 0.999879 | 0.471518 | 0.975507 | 0.091025 | 0.468234 | 0.221518 | 0.567425 |
(C46H90NO10P, +H) | -0.158368 | -0.158368 | -0.146483 | -0.075452 | 0.844551 | -0.098018 | -0.146478 | -0.128755 | 0.270007 | -0.129845 | ... | 0.012548 | 0.118463 | -0.128715 | -0.186785 | -0.007145 | -0.145936 | 0.599686 | 0.371394 | 0.639227 | 0.209528 |
(C44H80NO8P, +K) | 0.712669 | 0.712669 | 0.634190 | 0.763761 | 0.545115 | 0.771138 | 0.634164 | 0.711596 | 0.866938 | 0.596540 | ... | -0.005017 | 0.777400 | 0.711613 | 0.557933 | 0.329890 | 0.634538 | 0.455223 | 0.700800 | 0.534026 | 0.815117 |
(C42H82NO8P, +Na) | 0.881005 | 0.881005 | 0.872070 | 0.896388 | 0.603782 | 0.838684 | 0.872061 | 0.908116 | 0.970683 | 0.823794 | ... | -0.001476 | 0.927534 | 0.908125 | 0.853167 | 0.469023 | 0.871687 | 0.423398 | 0.707348 | 0.523192 | 0.764953 |
(C37H71O8P, +Na) | 0.954757 | 0.954757 | 0.934630 | 0.978277 | 0.311323 | 0.830642 | 0.934629 | 0.971658 | 0.857565 | 0.884022 | ... | -0.007087 | 0.879542 | 0.971660 | 0.932100 | 0.418883 | 0.934401 | 0.192368 | 0.534684 | 0.378283 | 0.689998 |
(C40H79O10P, +Na) | 0.999347 | 0.999347 | 0.951841 | 0.966621 | 0.237472 | 0.830904 | 0.951840 | 0.981573 | 0.842813 | 0.922001 | ... | -0.007330 | 0.850054 | 0.981564 | 0.933843 | 0.379390 | 0.951060 | 0.144795 | 0.482258 | 0.282528 | 0.630409 |
(C46H84NO8P, +K) | 0.598949 | 0.598949 | 0.541537 | 0.656881 | 0.633622 | 0.766413 | 0.541488 | 0.615919 | 0.814744 | 0.505041 | ... | -0.001309 | 0.726755 | 0.615941 | 0.479559 | 0.389002 | 0.540876 | 0.567930 | 0.670072 | 0.565501 | 0.743202 |
(C28H33O14, +Na) | 0.025906 | 0.025906 | 0.013698 | 0.039182 | 0.048013 | 0.029408 | 0.013688 | 0.034824 | 0.030510 | 0.046188 | ... | -0.001915 | 0.066998 | 0.034829 | 0.028291 | 0.222509 | 0.012846 | 0.017974 | 0.013835 | 0.053694 | -0.017291 |
(C45H91N2O6P, +K) | -0.078443 | -0.078443 | -0.090400 | -0.068159 | 0.138939 | -0.060259 | -0.090502 | -0.062721 | -0.018367 | -0.045871 | ... | -0.002302 | -0.000804 | -0.062710 | -0.073268 | 0.677184 | -0.091331 | 0.070271 | 0.066088 | 0.131005 | -0.071368 |
(C46H84NO8P, +H) | 0.374061 | 0.374061 | 0.394577 | 0.436341 | 0.954995 | 0.466528 | 0.394545 | 0.427361 | 0.741312 | 0.382491 | ... | 0.007937 | 0.629565 | 0.427395 | 0.365127 | 0.402726 | 0.394267 | 0.679322 | 0.667131 | 0.688433 | 0.572273 |
(C41H82NO8P, +H) | 0.938958 | 0.938958 | 0.888678 | 0.932395 | 0.197604 | 0.797989 | 0.888675 | 0.935026 | 0.777460 | 0.948548 | ... | -0.007908 | 0.914281 | 0.935018 | 0.897701 | 0.361409 | 0.888487 | 0.058150 | 0.441738 | 0.209431 | 0.617771 |
(C41H83N2O6P, +Na) | 0.790232 | 0.790232 | 0.750700 | 0.794648 | 0.335099 | 0.979850 | 0.750699 | 0.829114 | 0.781973 | 0.715908 | ... | -0.005648 | 0.779169 | 0.829108 | 0.757378 | 0.343047 | 0.747780 | 0.348768 | 0.454985 | 0.335521 | 0.583238 |
(C42H82NO8P, +H) | 0.617407 | 0.617407 | 0.600632 | 0.612815 | 0.753103 | 0.571425 | 0.600625 | 0.637339 | 0.804308 | 0.721005 | ... | 0.006482 | 0.813286 | 0.637345 | 0.590777 | 0.354026 | 0.599305 | 0.440937 | 0.609404 | 0.509301 | 0.556482 |
(C46H80NO8P, +K) | 0.610930 | 0.610930 | 0.657352 | 0.659183 | 0.597602 | 0.491470 | 0.657343 | 0.628072 | 0.838353 | 0.517155 | ... | -0.002591 | 0.699062 | 0.628089 | 0.562409 | 0.462758 | 0.659030 | 0.410232 | 0.951021 | 0.553891 | 0.907534 |
(C37H68O4, +H) | 0.680584 | 0.680584 | 0.684260 | 0.791282 | 0.715124 | 0.670272 | 0.684249 | 0.710134 | 0.897573 | 0.616228 | ... | 0.001152 | 0.866172 | 0.710160 | 0.639643 | 0.390156 | 0.687006 | 0.505569 | 0.758991 | 0.696927 | 0.851164 |
(C39H75O8P, +K) | 0.877262 | 0.877262 | 0.934213 | 0.925133 | 0.472458 | 0.738031 | 0.934212 | 0.896959 | 0.907352 | 0.783411 | ... | -0.003472 | 0.852741 | 0.896970 | 0.892038 | 0.490283 | 0.935419 | 0.330791 | 0.710169 | 0.520290 | 0.811476 |
(C42H82NO6P, +K) | -0.057401 | -0.057401 | -0.071163 | -0.047388 | 0.066776 | -0.040896 | -0.071303 | -0.044335 | -0.032253 | -0.029844 | ... | -0.001480 | 0.000079 | -0.044328 | -0.052903 | 0.682871 | -0.072057 | 0.030825 | 0.043389 | 0.112792 | -0.068358 |
(C26H30O12, +Na) | 0.010865 | 0.010865 | -0.001546 | 0.025708 | 0.026594 | 0.017037 | -0.001561 | 0.018531 | 0.014167 | 0.026144 | ... | -0.001465 | 0.050583 | 0.018534 | 0.011355 | 0.186877 | -0.002215 | 0.005312 | 0.005111 | 0.039767 | -0.017069 |
(C39H79N2O6P, +K) | -0.023062 | -0.023062 | -0.050788 | -0.019007 | 0.115662 | 0.058220 | -0.050867 | -0.003415 | 0.014955 | 0.005829 | ... | -0.002467 | 0.041734 | -0.003408 | -0.025108 | 0.598397 | -0.052413 | 0.101190 | 0.012588 | 0.146437 | -0.080461 |
(C39H73O8P, +K) | 0.668030 | 0.668030 | 0.673405 | 0.760046 | 0.786346 | 0.662500 | 0.673399 | 0.688299 | 0.935355 | 0.568486 | ... | 0.001652 | 0.810951 | 0.688329 | 0.599187 | 0.388371 | 0.675261 | 0.612481 | 0.824019 | 0.758521 | 0.870343 |
(C48H91NO8, +K) | -0.158368 | -0.158368 | -0.146483 | -0.075452 | 0.844551 | -0.098018 | -0.146478 | -0.128755 | 0.270007 | -0.129845 | ... | 0.012548 | 0.118463 | -0.128715 | -0.186785 | -0.007145 | -0.145936 | 0.599686 | 0.371394 | 0.639227 | 0.209528 |
(C10H7NO3, +K) | 0.427481 | 0.427481 | 0.428150 | 0.554429 | 0.796830 | 0.470626 | 0.428135 | 0.455527 | 0.724911 | 0.369360 | ... | 0.008353 | 0.604125 | 0.455564 | 0.372570 | 0.326230 | 0.428474 | 0.671104 | 0.654067 | 0.884128 | 0.674531 |
(C20H38O2, +H) | -0.007426 | -0.007426 | -0.004923 | -0.006511 | 0.008136 | -0.004867 | -0.004923 | -0.007160 | 0.000489 | -0.000408 | ... | 1.000000 | -0.002736 | -0.007158 | -0.006625 | 0.000142 | -0.005837 | 0.006002 | 0.007444 | 0.008340 | -0.005509 |
(C43H84NO8P, +H) | 0.851782 | 0.851782 | 0.818077 | 0.879146 | 0.491154 | 0.801050 | 0.818067 | 0.859451 | 0.889056 | 0.863759 | ... | -0.002736 | 1.000000 | 0.859456 | 0.809514 | 0.407435 | 0.818763 | 0.276569 | 0.625656 | 0.394536 | 0.744101 |
(C42H78NO8P, +H) | 0.982674 | 0.982674 | 0.958749 | 0.959580 | 0.282133 | 0.841924 | 0.958748 | 0.999993 | 0.856479 | 0.915163 | ... | -0.007158 | 0.859456 | 1.000000 | 0.955863 | 0.427685 | 0.957441 | 0.168933 | 0.517213 | 0.306024 | 0.645578 |
(C44H82NO8P, +H) | 0.933620 | 0.933620 | 0.976383 | 0.900842 | 0.213727 | 0.760261 | 0.976380 | 0.955873 | 0.780084 | 0.896570 | ... | -0.006625 | 0.809514 | 0.955863 | 1.000000 | 0.477581 | 0.975415 | 0.091060 | 0.468987 | 0.222420 | 0.567233 |
(C43H87N2O6P, +K) | 0.382377 | 0.382377 | 0.477978 | 0.381378 | 0.259783 | 0.308824 | 0.477895 | 0.427682 | 0.430843 | 0.369670 | ... | 0.000142 | 0.407435 | 0.427685 | 0.477581 | 1.000000 | 0.476365 | 0.169598 | 0.500062 | 0.268338 | 0.374949 |
(C42H83O10P, +Na) | 0.952055 | 0.952055 | 0.998177 | 0.929519 | 0.265818 | 0.766656 | 0.998177 | 0.957449 | 0.843408 | 0.876900 | ... | -0.005837 | 0.818763 | 0.957441 | 0.975415 | 0.476365 | 1.000000 | 0.154454 | 0.561858 | 0.289387 | 0.669347 |
(C8H20NO6P, +K) | 0.152810 | 0.152810 | 0.155859 | 0.212115 | 0.740538 | 0.340360 | 0.155857 | 0.168905 | 0.501314 | 0.058189 | ... | 0.006002 | 0.276569 | 0.168933 | 0.091060 | 0.169598 | 0.154454 | 1.000000 | 0.473728 | 0.738939 | 0.402623 |
(C48H84NO8P, +K) | 0.490398 | 0.490398 | 0.561101 | 0.543898 | 0.636748 | 0.448071 | 0.561080 | 0.517190 | 0.777492 | 0.408694 | ... | 0.007444 | 0.625656 | 0.517213 | 0.468987 | 0.500062 | 0.561858 | 0.473728 | 1.000000 | 0.567532 | 0.863574 |
(C16H16O11S, +K) | 0.289137 | 0.289137 | 0.287036 | 0.413908 | 0.721979 | 0.326168 | 0.287023 | 0.305991 | 0.593308 | 0.193200 | ... | 0.008340 | 0.394536 | 0.306024 | 0.222420 | 0.268338 | 0.289387 | 0.738939 | 0.567532 | 1.000000 | 0.559128 |
(C45H78NO8P, +K) | 0.636388 | 0.636388 | 0.665877 | 0.725554 | 0.514660 | 0.593814 | 0.665875 | 0.645559 | 0.833599 | 0.515406 | ... | -0.005509 | 0.744101 | 0.645578 | 0.567233 | 0.374949 | 0.669347 | 0.402623 | 0.863574 | 0.559128 | 1.000000 |
51 rows × 51 columns
[ ]: